Last time I checked the lifetimes of different types of services and want to share with you my findings.
Application
Having such controller:
[ApiController] [Route("[controller]")] public class FooController : ControllerBase { private ISingletonService _singleton1; private ISingletonService _singleton2; private IScopedService _scoped1; private IScopedService _scoped2; private ITransientService _transient1; private ITransientService _transient2; public FooController( ISingletonService singleton1, ISingletonService singleton2, IScopedService scoped1, IScopedService scoped2, ITransientService transient1, ITransientService transient2) { _singleton1 = singleton1; _singleton2 = singleton2; _scoped1 = scoped1; _scoped2 = scoped2; _transient1 = transient1; _transient2 = transient2; } [HttpGet] [Route("Singleton")] public List<string> Singleton() { return new List<string> { _singleton1.GetGuid(), _singleton2.GetGuid() }; } [HttpGet] [Route("Scoped")] public List<string> Scoped() { return new List<string> { _scoped1.GetGuid(), _scoped2.GetGuid() }; } [HttpGet] [Route("Transient")] public List<string> Transient() { return new List<string> { _transient1.GetGuid(), _transient2.GetGuid() }; } }
and such startup (full repo address in the end of post)
public void ConfigureServices(IServiceCollection services) { services.AddTransient<ITransientService, TransientService>(); services.AddScoped<IScopedService, ScopedService>(); services.AddSingleton<ISingletonService, SingletonService>(); services.AddControllers(); }
I queried each endpoint (singleton, scoped, transient) two times. Here are the results:
Singleton
first request
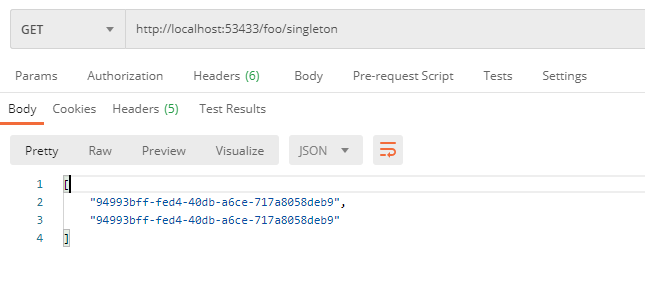
second request
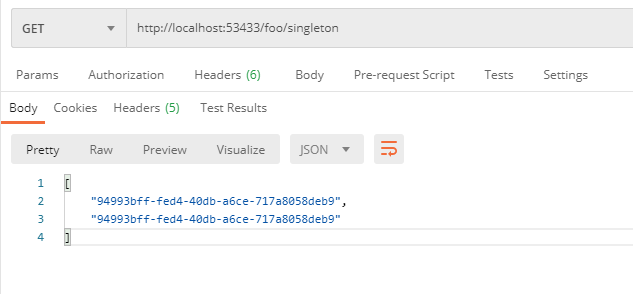
Conclusion
- doesn’t matter how many times you request endpoint. There is only one instance.
Singleton service is created
- first time they’re requested and is not destroyed
- by developer
When useful?
- caching
- configuration
- global rules
Scoped
first request
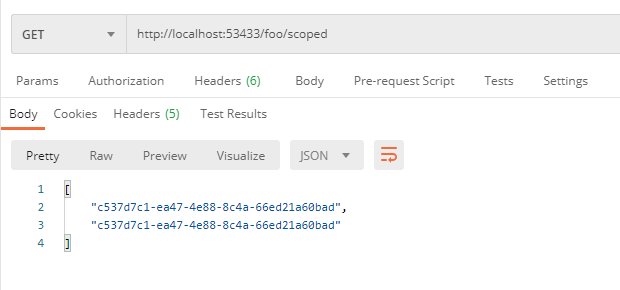
second request
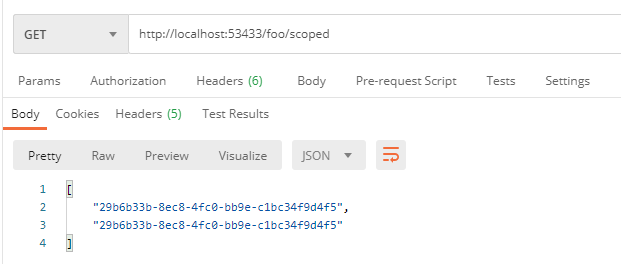
Conclusion:
- each request generates new Guid
- same instance is shared in the boundary of single request
each time you access the page the service is createdwhen useful?
- persist state throughout application per request
Transient
first request
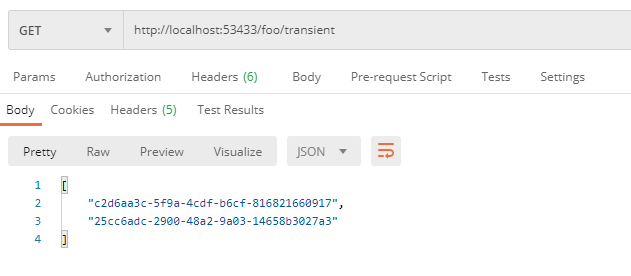
second request
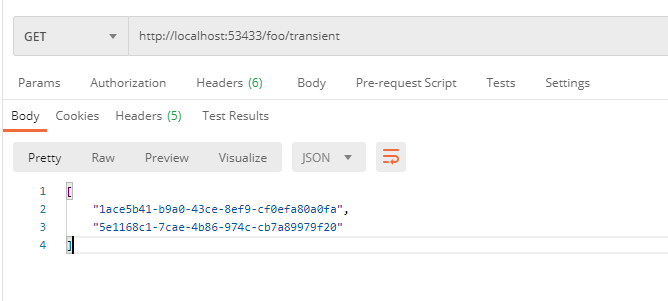
Conclusion
- each request generates new Guids
- new Guid (new instance) of service is created on each access to instance
Transient service is created
- each time they’re requested from the service container
When useful?
- weightless stateless services
Link to the repo: https://github.com/simplyaboutcode/ServiceLifetime